Bookmark AJAX pages.
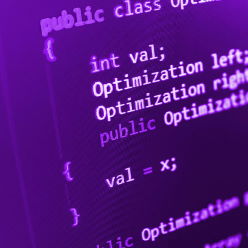
Javascript can modify and read this value with no refresh of the page. Also, if you have anchors in your page, like... <a name="hash" Javascript code like
window.location.hash='hash';will scroll the page there.
Multiple IE versions on the same computer
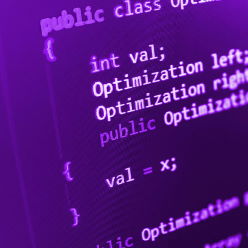
Usually, when I decide to blog on something, I do the testing and researching and installing first, then blog about it. But now I intend to use this cool site:
http://tredosoft.com/Multiple_IE
which boasts with installing all IE versions since 3.0 (oh, beloved 3.0) on the same computer without any problems. Since I am not a trusty guy, I blog about it before, then, if no one ever hears from me again, it means that no browser on any computer worked anymore after this :)
Well, without further ado, let me proceed :-SS
Extra info: http://www.positioniseverything.net/articles/multiIE.html
Step 1: Installing IE7.
Of course I had to validate my copy of Windows to download it, then I had to download all updates (even if I went to Windows Update right before installing IE7), then wait until it searched my computer for malicious software, then installing everything. You can't imagine a smoother installer. It just tells you to wait and does everything in the background, showing you meaningless text labels, a cool progress bar and, of course, asking you to close everything before and restart Windows after the installation.
But it worked, and I am not writing this from Firefox :)
Step 2: Installing multiple-ie-setup.exe
Wow! It took around 1 minute to install everything. Of course, not everything is going as smoothly as planned. First of all, I can't see Blogger (and suposedly not any other cookie using site) in IE6.0. Then, it redirects me to a nocookies.html files that doesn't exist :-/ But that's a Blogger issue. The Options menu in IE6.0 is actually the IE7.0 menu and the settings for cookies cannot be overriden. Actually, you can, but the settings won't save.
After looking at the TrendoSoft site, I've noticed that this bug is considered solved, even if some of the people seem to continue to have problems. So I've tested more throughly. Session variables seems to be saved, but Blogger continues to take me to the nocookies page. Also, AjaxPro, the ajax library I am using, doesn't seem to work with IE 6.0.
All in all it seems a pretty functional program. However, the type of sites that I am building have certain characteristics that seem not to work with it. I will try to log on TrendoSoft and get the problem fixed, but I guess Yousif did the best he could so far and resolving every issue I have will be hard if not impossible.
Javascript replace function
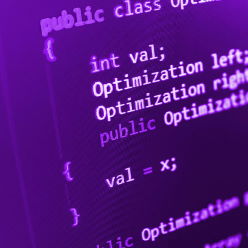
Simon Willison's Weblog contains a good article on this.
Basically, if you use str1.replace(str2,str3) it will return str1 with the first occurence of str2 replaced with str3. If you use str1.replace(regexp1,str3) and the regular expression has the g modifier it will return str1 with all matches of regexp1 replaced with str3.
A regular expression looks like : /searchpattern/modifiers.
You can create a regular expression from a string by using the
new RegExp(str2,modifiers)syntax.
The problem comes when you want to create a regular expression from a variable that may contain regular expression escape sequences. Here is Simon Willison's function that "escapes" the string in order to use the RegExp syntax safely, slightly modified to contain the '^' character:
RegExp.escape = function(text) {
if (!arguments.callee.sRE) {
var specials = [
'/', '.', '*', '+', '?', '<', '>',
'(', ')', '[', ']', '{', '}', '\\', '^'
];
arguments.callee.sRE = new RegExp(
'(\\' + specials.join('\\') + ')', 'g'
);
}
return text.replace(arguments.callee.sRE, '\\$1');
}
Example: str=str.replace(/\\/g,'\\\\') (replace slashes with double slashes)
Checking your web app against different browser resolutions
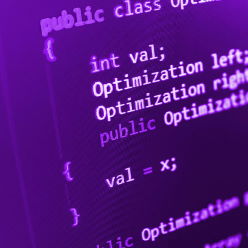
- I have found three basic methods:
- Use an online type of viewer.
You select the resolution, type in the URL of your site, then sit back and relax.- I see a lot of problems for this method:
- you don't want everybody to know you are working on a site
- you don't want the site to be open to the public while you work on it
- you need an internet connection
- Use an external program.
BrowserSizer is as good as any. It's free and easy to use. You select the resolution and it resizes your IE window accordingly.
The only problem I see is that you need the program. You install it, it messes with your browser settings, etc. - Use a javascript script in the IE addressbar:
javascript:db=document.body;bst=db.style.zoom=1;rd=prompt("Width:",800);
db.style.zoom=db.offsetWidth/rd;void('')
Unfortunately, the zoom messes up quite a few things, including fixed table headers or custom javascript controls. However, I do believe it is the best solution for most situations.
Programatically trigger event in Javascript
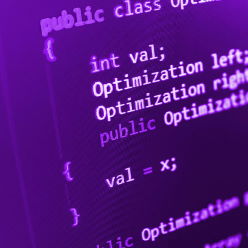
IE way) really simple, use element.fireEvent(eventName,[eventObject]), where element is a HTML element and eventName is something like "onclick";
DOM way) really complicated. A short example:
function simulateClick() {
var evt = document.createEvent("MouseEvents");
evt.initMouseEvent("click", true, true, window,
0, 0, 0, 0, 0, false, false, false, false, 0, null);
var cb = document.getElementById("checkbox");
var canceled = !cb.dispatchEvent(evt);
if(canceled) {
// A handler called preventDefault
alert("canceled");
} else {
// None of the handlers called preventDefault
alert("not canceled");
}
}
As you can see, you need to create the event, then initialize it, then dispatch it.
- Things you need to take care with:
- in the DOM model the event name is not prefixed with "on". In the IE way, the event is prefixed with "on". ex: DOM:click IE:onclick.
- in the DOM model the event triggering does everything the event would have done, like setting a value, in IE it does not. ex: triggering onclick in the above example would check or uncheck the box. In IE you need to change the value yourself.
This is code I did to programatically click a checkbox and also trigger the onclick event:
if (box.onclick)
{
if (box.dispatchEvent) {
var clickevent=document.createEvent("MouseEvents")
clickevent.initEvent("click", true, true)
box.dispatchEvent(clickevent)
} else
if (box.fireEvent) {
box.checked=!box.checked;
box.fireEvent('onclick');
} else
box.onclick();
} else box.checked=!box.checked;
Add table header on each printed page
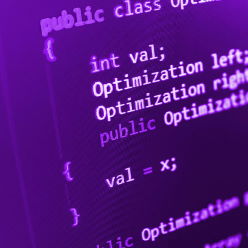
There is a simple solution for printing tables with repeating headers on each printed page. It involves CSS styling of the THEAD section of a table. Unfortunately, neither DataGrids nor GridViews render the THEAD tag. Somehow, Microsoft seems hellbent against it. So either create a control that renders THEAD, then add "display:table-header-group;" to the THEAD style, or use this Javascript function:
function AddTHEAD(tableName)
{
var table = document.getElementById(tableName);
if(table != null)
{
var head = document.createElement("THEAD");
head.style.display = "table-header-group";
head.appendChild(table.rows[0]);
table.insertBefore(head, table.childNodes[0]);
}
}
Update:Building a GridView, DataGrid or Table with THEAD, TBODY or TFOOT sections in NET 2.0
CSS Style Sheets Fundamentals
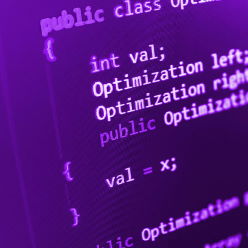
1. CSS Style Sheets are good. Because you can change a lot from the file without changing the site. One could even imagine a complete site makeover only from the style. Remember that style can have color, font size, div position, borders, anything one can think of and it's not in Javascript :)
2. As a corolary to the first rule, style tags and color attributes and stuff like that inside the html file are NOT good. A colleague of mine has submited the idea that there are exceptions to this, like horizontal alignment in table columns and similar basic things. But I say NO! :) If there was a requirement for a certain column to be aligned differently, then it belongs to another style class, even if it's only one column in a thousand columns with the same alignment. I do admit that it's difficult and annoying to copy all the attributes of a class to another just to change something as trivial as the text color. Which brings me to point 3.
3. You can't nest or inherit CSS classes, which is really dumb. I mean, I want a base class to contain text font and color and everything and I want to create another class that says "I am like class1, but with bold font". You can't do that as far as I know, but you can combine classes. An element can have a class="redFont blueBoldFont" which will combine the two CSS classes, and they will overwrite each other. In this case the font will be blue and bold, as the blue color will override the red.
4. CSS classes can be selective. For example .redFont td { color:red; } will apply only to table cells within elements with the redFont class. That is different from the td.redFont notation, which tells what will happen with table cells that have the redFont class. I would suggest avoiding this type of notation if possible. Also, one can somewhat separate the behaviour of a class inside another class: .redFont .blueBoldFont { color:magenta; } in a css file or within a style tag will signify that the blueBoldFont class within the redFont class will have magenta color. Everything else being a combination of the two mentioned classes. This is a little akward, but it works. Warning! the notation .class1.class2 works in Internet Explorer only. The proper notation is the classes names preceded by a dot and separated by spaces.
5. One cannot configure custom html attributes or javascript events in style. That is a hinderance, but I don't know if adding it would have helped things. I mean, I do want to configure all the elements having a draggable class to have special mousedown and mouseup events, but this can also be done from javascript.
What does this mean? It means that a well designed site will have ALL the styling, even the position and size of movable elements, in a CSS file, as it will have all the Javascript in a JS file. This kind of file separation insures that the styling can be changed independently from the Javascript code and the HTML source. It also means that when designing a Web Control, creating a complicated style system for all its elements is not necessary. Just give a default class to each pertinent html element and then give the entire control a single configurable CSS class. Then combine classes to style each element.
Internet Explorer and the hidden table cells.
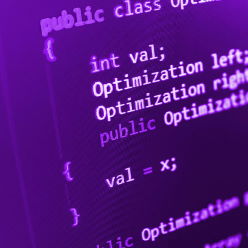
More detailed, style.display='none' makes the cellIndex of the next cells to decrement, while style.visibility='hidden' does not decrement the cellIndex, but keeps the formatting of the page, so you actually see a big empty space where the cell used to be.
I wouldn't have mind this if the Microsoft guys used another index to show the internal value, but they didn't! I have no idea what is the internal index of my cells anymore! Grrr!
The Microsoft Developer Toolbar
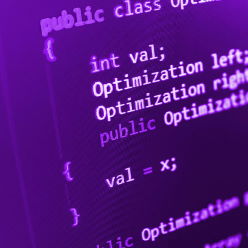
Internet Explorer Developer Toolbar
Then go in Internet Explorer and add the Developer toolbar. It is very nice
for web developers and javascript programmers
Also, check this out:
Code Spy